Extending K8S API
- Lency Korien
- Oct 10, 2024
- 3 min read
Kubernetes isn’t just about managing those swanky Pods and Services; it’s like a LEGO set, and with the right pieces, you can build pretty much anything.
Enter the world of Custom Resource Definitions, or CRDs for the cool kids. Imagine being able to teach your Kubernetes new tricks without waiting for the next big release. That’s exactly what CRDs let you do!
In this blog post, we will explore how to extend the Kubernetes API with Custom Resource Definitions (CRDs).
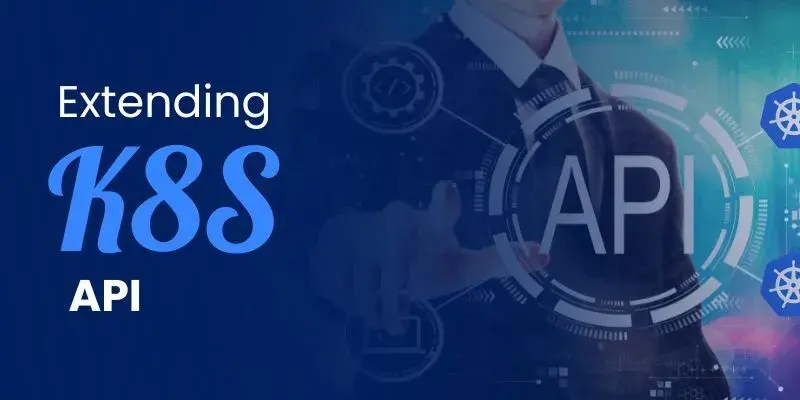
Topics To be covered :
Registering a Custom API in Golang
Creating a Custom Resource Definition ( CRD ) to Register our Custom Resource
A Look at the General API of Kubernetes Resources
1. The Basics: API Groups and Versions
API Groups: To make its API more extensible and modular, Kubernetes has different API groups like core, apps, batch, extensions, and more. These groups represent various functionalities and components.
Versions: Each group can have multiple versions, typically marked as v1, v1beta1, etc. This is because the Kubernetes API evolves over time, and different versions can coexist.
2. Resources and Kinds
Resources: These are a persistent entity in the Kubernetes system. Pods, Services, and Nodes are all examples of resources.
Kinds: This denotes the type of the resource. When you create or read a resource, you work with its kind. For instance, the kind for a Pod is Pod, and for a Service, it’s Service.
3. Object Metadata Every Kubernetes resource comes with metadata. This section usually includes details like:
name: Unique within a namespace, used to identify the resource.
namespace: Helps with the resource’s scope. Some resources are cluster-scoped (like Node), while others are namespace-scoped (like Pod).
labels and annotations: Key-value pairs that you can attach to resources for various purposes, from organizational patterns to notes.
4. Spec and Status Almost every Kubernetes resource has these two main fields:
Spec (Specification): This is what you provide. It’s your desired state for the resource.
Status: This is what Kubernetes provides back. It tells you the current state of the resource and how it compares to the spec.
5. API Endpoints For every resource, there are specific API endpoints. These are the URLs you’d hit if you were to interact directly with the Kubernetes API server. For instance, /api/v1/namespaces/{namespace}/pods/{pod} would be an endpoint for a specific pod.
Registering a Custom API in Golang
Before you can use a custom resource in Kubernetes, you need to define it, usually in Golang. Here is how you can do it.
Define your types in Go. Use tags to define how the fields are serialized in JSON or YAML.
package v1
type MyCustomResourceSpec struct {
Field1 string `json:"field1,omitempty"`
Field2 int `json:"field2,omitempty"`
}
type MyCustomResource struct {
metav1.TypeMeta `json:",inline"`
metav1.ObjectMeta `json:"metadata,omitempty"`
Spec MyCustomResourceSpec `json:"spec,omitempty"`
Status MyCustomResourceStatus `json:"status,omitempty"`
}
type MyCustomResourceList struct {
metav1.TypeMeta `json:",inline"`
metav1.ListMeta `json:"metadata,omitempty"`
Items []MyCustomResource `json:"items"`
}
Next, we must create a deep copy to make our API object compatible with the k8s object. Since the Object Interface requires DeepCopyObject()
type Object interface {
GetObjectKind() schema.ObjectKind
DeepCopyObject() Object
}
To generate a deepcopy we would use controller-gen run the below command to install at $GOPATH/bin/
We need to put kubebuilder tags to tell the controller-gen which object we need to create deecopy. The tag we use is // +kubebuilder:object:root=true as suggested here. We need to put this tag on MyCustomResource and MyCustomResourceList to generate deepcopy for that as given below. This would generate a file zz_deepcopy.go in the same directory.
You can check more info about: K8S API.
コメント